My first goal was just to generate a spiral of numbers. It wasn’t too difficult to code a two dimensional array, find the center and start plotting numbers in the appropriate cell. My app builds spirals up and to the right, but it would be quite simple to have it go a different direction.
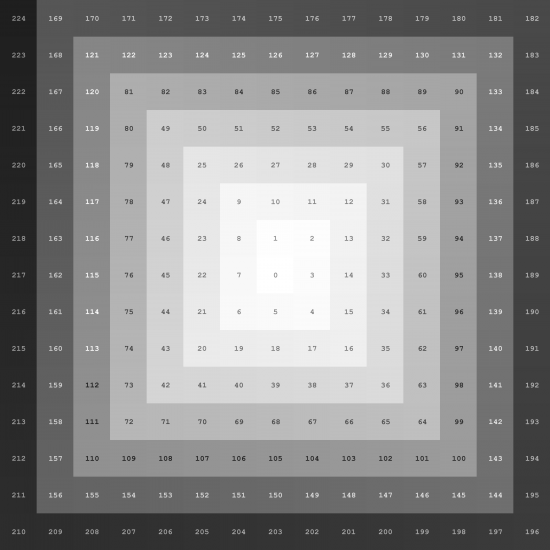
As an example, a 3×3 array after being filled with the spiral can be imagined like this:
8 1 2
7 0 3
6 5 4
5×5:
24 9 10 11 12
23 8 1 2 13
22 7 0 3 14
21 6 5 4 15
20 19 18 17 16
Ok, so this isn’t that interesting so far. So I decided to have it plot repeating sequences instead of just counting up endlessly. For example, I could plot 012 over and over and my 3×3 would then look like this:
2 1 2
1 0 0
0 2 1
5×5 with 01234 as sequence:
4 4 0 1 2
3 3 1 2 3
2 2 0 3 4
1 1 0 4 0
0 4 3 2 1
When I got to this point, I could tell something interesting was happening with the numbers, but I couldn’t really pinpoint it. I could tell there was some repeating patterning going on, but I couldn’t see it. So I wrote a little code to color code the number cells.
For example, coloring all the cells with a 0 one color and all the cells with a 1 another. Ok, so I did a little more than color a cell. I used the php gd libraries to build an image, colored each of the cells with a shade of grey (0 = lightest to highest number = black) and then added some options to allow me to write the values into the cells as text or just leave them off.
Here’s an example of a 5×5 grid with a five element sequence where the values have been written into each cell.
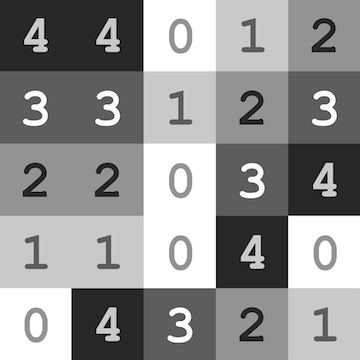
Let’s take a look at what happens with different sequences. These are all 11×11 grids. Starting on the left, we plot a two digit sequence, then three and so on up to a ten digit sequence on the far right.

Here they are again without the numbers:

Coding more blocks into the array effectively “zooms out” on the spiral. Here are the same sequences at 21×21:

And again at 51×51:

Thanks for playing!
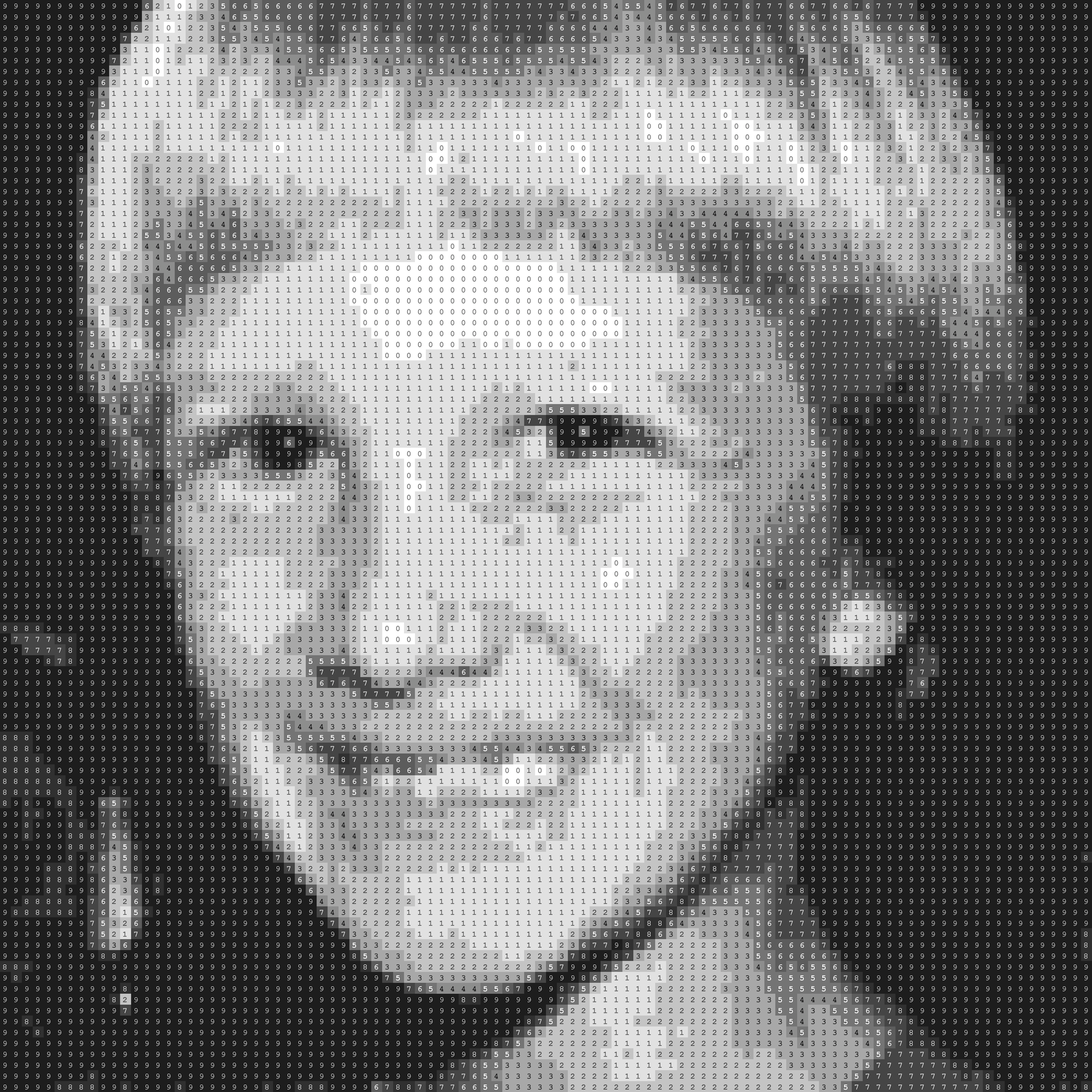
This looks really cool. It reminds me of "Ulam's Spiral" (which has also been done with hexagonal grids, too.. something you could try here), and also a bit like certain kinds of "cellular automata". Although this couldn't be described as cellular automata (there is no evolution of the system), it does share the interesting outcome of a simple set of rules leading to surprising complexity.
Would you be willing to share the code for this? Maybe toss it up on Github or somewhere?
Not in it's current state. But I'd be willing to clean it up and do so. 🙂 Thanks for the new leads on ideas.
It is really cool.
@jbury Ulam’s Spiral is what the Vi Hart videos explore.
What library did you use to create the images?
Oh, and I wonder what would happen if you did one spiral with one period in one color, overlaid with another spiral with a different (probably relatively prime) period in another color. If converted to gray scale, it would look like a spiral with period equal to the least common multiple of the two periods, but it would also show “harmonic” features in the color version – it would show how the two periods interact.