A great skill a Salesforce administrator can have in his or her back pocket is basic knowledge of the Salesforce Apex programming language. This awareness empowers administrators to better understand the behavior of an application. As a result, administrators can explain underlying logic to users as well as diagnose issues without having to go to a Salesforce developer for help.
To help Salesforce administrators explore learning Apex coding, I recommend writing a Hello World program. In this post, I will walk you through it. In the process, you will learn a few simple but useful programming concepts: variables, primitives, conditionals, and methods.
Using the Developer Console
Before we can start coding our Hello World program, we need to learn how to run it. There are several ways to execute Apex coding and programming on the Salesforce platform. The simplest one is using the Developer Console to execute code in an anonymous block. Let’s start by opening the console.
- Make sure your profile has the “Author Apex” permission enabled.
- Click on the gear icon, then select Developer Console. If you are in classic mode, click on your name on the top right, then select Developer Console.
- Once the Developer Console loads, click debug on the menu and select Open Execute Anonymous Window. You can also use the shortcut CTRL+E.
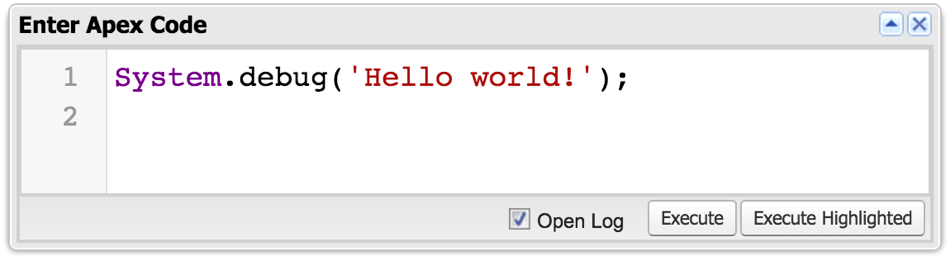
- Type the following line of code:
System.debug('Hello world!');
- Check the Open Log option.
- Click Execute.
- Once the Execution Log loads, check the Debug Only checkbox.
- You should see your Hello world message. Congratulations, you just wrote your first Salesforce Apex program!
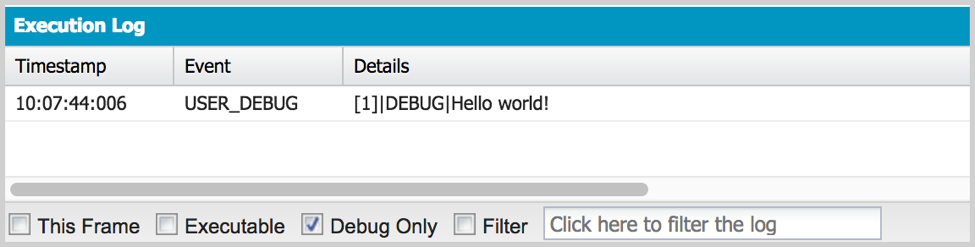
Variables in the Salesforce Apex Programming Language
For our next step, let’s try something a little more interesting. We will use a variable and assign “Hello World!” to it. Then, we will output the value of that variable to the debug log.
- Open the Execute Anonymous Window from the debug menu or using the shortcut, CTRL+E.
- Modify your code to look like this:
String message = 'Hello world!';
System.debug(message);
- Click Execute.
- Once the Execution Log loads, check the Debug Only checkbox.
Notice the output is the same as the previous time we ran the code. Line 1 has two purposes — one is declaring the variable, which tells Apex we want to create a new variable of type String. The second purpose is to set its value to “Hello world!”.
An interesting property of variables is that they are mutable. That means we can modify their content after they are created. Let’s try it. Update the code again and add one more line.
String message = 'Hello world!';
message = 'Hi there!';
System.debug(message);
Overwriting Your Values
Run the code again following the same steps as before. This time you will see “Hi there!”, but “Hello World!” will not be displayed. Let’s take a look at what just happened. In line 1, we created a variable called message and assigned a value of “Hello World!” to it. Then, in line two, we assigned a new value to it, “Hi there”. The new value took the place of the old one, overwriting it.
You can also perform operations on variables using the Salesforce Apex programming language. In this case, since we are dealing with Strings, we can do things like concatenation (stitching two or more strings together) or changing the casing to all caps.
String greeting = 'Hello';
String who = 'world';
who = who.toUpperCase();
String message = greeting + ' ' + who + '!';
System.debug(message);
When you run this code, you will see it generates a similar output as the first example. That is because we are concatenating a variable called greeting which contains the word “Hello”, a space, the who variable containing “WORLD”, and the exclamation point symbol. Those four things together form the phrase “Hello WORLD!”.
Are you wondering why the word “world” is all in capitals? That is because of line 3 where we call the toUpperCase() function which gives us back the same string but with all its letters capitalized.
Primitive Types in the Salesforce Apex Programming Language
So far, we have only used variables of type String in our examples. String variables allow you to store a collection or characters, numbers or symbols but there are other types that we can use for different purposes. For example, Integer can be used for storing whole numbers, or Double for numbers with decimals. The Salesforce Apex programming language provides 12 primitive types but you can also create custom types by writing your own classes.
List of Salesforce Apex Primitives
Blob | Binary Data |
Boolean | The values true or false |
Date | A calendar date |
Datetime | A calendar date and time |
Decimal | A number that includes a decimal point |
Double | A double precision floating point number |
ID | A 15 or 18-digit Salesforce ID |
Integer | A whole number — between -2,147,483,648 and 2,147,483,647 |
Long | A large whole number — between -263 and 263-1 |
Object | A generic type that can store a value of any other type |
String | Any set of characters — surrounded by single quotes |
Time | A time |
Conditional Statements in Salesforce Apex Programming
In order to have your code make decisions and behave differently based on the value of a variable (or user input), you need to use a conditional statement. The most common type of conditional is the if-else statement, which is very similar to the IF() function in Salesforce formulas. Just like its formula counterpart, it has three parts: the logical test, the true block, and (optionally) the false block. If the logical test evaluates to true, the true block is executed, otherwise, the false/else block is. A code block is one or more lines of code wrapped with curly brackets.
Boolean formal = true;
String who = 'world';
String greeting;
if (formal == true) {
greeting = 'Hello';
} else {
greeting = 'Hi';
}
String message = greeting + ' ' + who;
System.debug(message);
When you run this code, you will see that it outputs “Hello world”. The logical test was checking if the variable formal was equal to true. It, therefore, executed the first code block (line 5) which sets the variable greeting equals to “Hello”. If it had not been true, it would have executed the false/else block (line 7) which would have set the greeting variable to “Hi”. You can try running it again, but setting the variable formal equals to false on line 1.
Writing Methods in the Salesforce Apex Programming Language
As a Salesforce administrator, you are probably already familiar with the concept of methods which are very similar to functions in validation rules and formula fields. We have also already used two methods in our examples. One was System.debug(), and the other one was String.toUpperCase(). You already know how to invoke them. Now let’s take a closer look.
Apex Methods
Methods represent a block of code that can be invoked (or called) from somewhere else. They are useful in many ways but have two main purposes: First, you can use methods to avoid repetition by writing your code once and calling it multiple times as needed. Second, methods make code cleaner and easier to read by allowing sections to be abstracted out.
Let’s modify our Hello Word program to move the greeting selection logic inside a method, which we will call getGreeting().
Boolean formal = true;
String who = 'world';
String greeting = getGreeting(formal);
String message = greeting + ' ' + who;
System.debug(message);
private String getGreeting(Boolean formal) {
if (formal == true) {
return 'Hello';
} else {
return 'Hi';
}
}
Now, if you want to quickly glance at the code, you should be able to tell at a high level what it does just by looking at the first 5 lines. If you are interested in the specifics of how the greeting is selected, you can look at lines 8 through 12 inside the method. This will make it easier for someone else to read your code or even for yourself, if in the future you want to refresh your memory on what the logic does.
This latest variation of the code will output “Hello world” just like previous versions but the order in which the lines get executed is a little different. First lines 1 and 2 will run. Once we get to line 3, in order to get the value to store in greeting, lines 6, 7, and 8 will get executed and getGreeting() will return “Hello”. Then execution continues on line 4, where “Hello world” will be stored in the message. Lastly, line 5 will be executed which will output the value of the message. At that point, the code is finished executing and terminates.
Further Reading on the Salesforce Apex Programming Language
Congratulations, you have reached the end of this tutorial. You should now be able to read and understand the gist of the code in your org, even if you don’t know exactly what each line does. You should also be able to make simple changes to existing code with the supervision of a more experienced developer.
If you enjoyed writing your first program and would like to learn more about the Apex programming language, you can take a look at these great resources:
- Trailhead – Gamified step-by-step guided tutorials on hundreds of topics
- Developer Console Basics – Trailhead module on using the Developer Console
- Apex Developer Guide – Official Apex programming documentation from Salesforce
- Primitive Data Types – Apex documentation about primitives
If need help using Salesforce Apex to customize your Salesforce solution, my team and I are happy to share our insights. Contact us today.