Note: read about the updates to Javascript and FileMaker 16 in an updated blog post here.
Have you heard of Carafe? Our free, open-source tool makes it easy to implement JavaScript in your FileMaker solution. Learn more.
JavaScript & FileMaker
FileMaker Inc. has released an update for FileMaker Pro 13 (see the release notes here). Among the list of improvements to FileMaker Pro and FileMaker Pro Advanced is the extended functionality of the FMP URL protocol to include support for calling a script to run in FileMaker from within a web viewer.
The FileMaker-Web Viewer communication is a big deal
Working with the web viewer is now a two-way street. You set the web viewer from FileMaker, and now you get data back from what is going on inside the web viewer.
Previously this capability was only available in FileMaker Go or if you happened to be running FileMaker Pro with a file hosted on FileMaker Server. By removing that constraint, this opens up a lot of possibilities for developers to extend and improve the solutions we build.
In this article, we will show why being able to communicate with a web viewer is a big deal.
The Challenge: Getting data out of the web viewer
With the web viewer, you can display nice, JavaScript-enabled functionality and have it sit side by side with our native FileMaker interface. Unfortunately, whatever you did in a web viewer remained sequestered in that web viewer; FileMaker had no way to know anything about it.
Until now, getting information from a web viewer and back into FileMaker has been a limiting factor. Now that you can utilize this functionality across the board, it’s much easier to invest time into learning how it works and how to use it in your solutions.
Hello World Example
We will begin with a simple script that shows a message displaying “Hello World” in a dialog. You can start with a new file or an existing file. Open scriptmaker by going to Scripts->Manage Scripts…
Create a new script and name it “Hello.” Add the script step Show Custom Dialog and specify the Title as “Hello” and the Message as “Hello World.” You can remove the cancel button and click “OK” and then save your script.
Now place a web viewer on the layout. We will simply display a link to click on. That’s it! Set the web viewer’s Web Address to the following:
"data:text/html,<html><body><a href=\"fmp://$/" & Get ( FileName ) & "?script=Hello\">" & "Hello World" & "</a></body></html>"
This is a data URL that displays all the html right inside the web viewer.
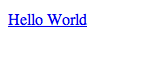
Save by clicking “OK” in the web viewer setup, then save your layout and enter Browse mode to test it out. Click the text that appears in the web viewer. You should see a dialog pop up, displaying our message.
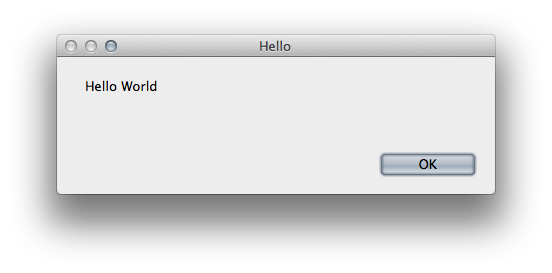
Let us break down what is happening. The URL you click on uses the fmp protocol. When you install FileMaker, the application registers this protocol with the operating system, telling it that when you launch a URL beginning with “fmp://” to hand it over to FileMaker to handle it.
The next part of that URL is a dollar sign ($) which references a file currently open by the application. We run the script from a currently open file via its own web viewer, which we can get with the function Get ( FileName ) in FileMaker. That way, it will always reference the currently open file we are in.
The rest of the URL (“?script=Hello”) tells FileMaker which script to run. You can also have a “param” value in your URL to also hand off a script parameter.
When you click that link, you launch FileMaker and telling it to run the script named “Hello” in a specified currently open file. We know the file is open because we were in that file when we clicked the link.
What else can we do with this? Adding a script parameter…
One example might be to collect input from an HTML form displayed in a web viewer. This should help you understand possibilities through the extension of your solutions in this way.
In FileMaker, you have no native option to have a password type input field unless you present the user with a custom dialog. If you want to have your password input field on a layout, you have limited options or must resort to script triggers and such.
In this example, we will use a web viewer to display an HTML form with our password type field. HTML has native support for this type of field, and when the form is submitted, hand off the value of that field to a FileMaker script as a parameter.
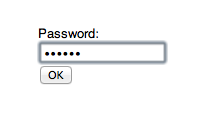
Create a script and name it “Password” and give it the message: “You entered the Password: “ & Get ( ScriptParameter )
"data:text/html," & "<html><body style="margin:4px; border:0; font: 10pt normal arial, sans-serif;">
</p>
<p>
Password:<br />
<form onsubmit="return submitForm()">
<input id="pass" type="password" /><br />
<input type="submit" value="OK" />
</form>
</p>
<p>
<script>
function submitForm() {
theParam = document.getElementById("pass").value;
theURL = "fmp://$/" & Get ( FileName ) & "?script=Password¶m=" + theParam;
window.location = theURL ;
document.getElementById("pass").value = "";
return false;
} </script>
</p>
<p>
</body></html>"
Note that all the quote marks in the html have been quoted with a backslash so FileMaker doesn’t stop there, and renders them in the HTML.
If familiar with HTML and javascript, you can see that we have a simple form that runs a javascript when submitted. The javascript references the input field via the ID we have given it and gets its value.
Then it builds the URL back to our FileMaker file. It calls the script we just created with the script parameter set to whatever we enter in our form.
Save it and go to browse mode. Enter some text in the password field, and you will see it automatically bullets the entry. Hit return or click the “OK” button and it will run our FileMaker script and show us what we entered.
Of course, we wouldn’t normally show someone their password. I just want to show how passing parameters can work.
Now something fancier
Now let’s have some fun. Javascript can do much more, and you can explore plenty of excellent publicly-available libraries and resources. I find Jquery, a favorite of mine, easy to work with.
For example, I found a resource for a color picker. Check it out here. So what if we want to have a color picker in our FileMaker layout that can set the color of our field to whatever color we pick?
For this example, I created a couple of fields to make it easier to type html and javascript. This means we won’t have to put a backslash in front of all the quote marks. FileMaker won’t evaluate this as part of its calculation and only include the text from our fields.
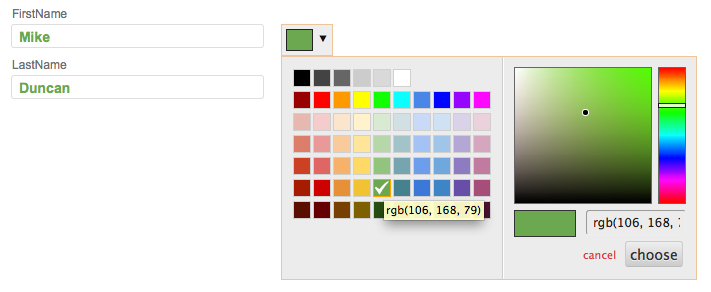
I have broken it up into two parts in order to include the part where FileMaker evaluates the file name of the current file and put all the pieces together to display my html in a web viewer.
"data:text/html," & resources::html1 & "fmp://$/" & Get ( FileName ) & "?script=SetRGB¶m=" + getRGB + "" & resources::html2
All the resources to drive this are referenced externally, so it loads in jquery along with the color picker javascript and CSS. This lightens the load of stuff that you have to build into FileMaker, but they could also be loaded locally if you want to take the time to do that. You might do that to run this example without an internet connection, but for this example, one is required, just like loading a web page.
In this example, we have added the fmp url to the callback function in jquery that gets run when a user selects a color. This hands off the RGB parameter selected and stores it in a field in FileMaker. The relevant part of javascript that is set, at runtime by FileMaker, in a callback function by jquery looks like this:
change: function() {
var getRGB = $("#full").spectrum("get");
window.location = "fmp://$/Javascript_Example?script=SetRGB¶m=" + getRGB + "";
},
Now, back in FileMaker, we can work with data that we have in our fields. An auto enter on the fields we want to change color, and we can parse out the RGB values we got back from the web viewer. We set its field definitions for auto enter like this:
Let ([
myField = Self;
values = Substitute ( RGB ; ["rgb("; ""] ; [")"; ""] ; [","; "¶"] );
red = GetValue ( values ; 1 );
green = GetValue ( values ; 2 );
blue = GetValue ( values ; 3 );
end = "" ] ;
Case (
IsEmpty (RGB); TextColorRemove ( myField ) ;
TextColor ( myField ; RGB ( red ; green ; blue ) )
)
)
Now untick the box that says “Do not replace existing value of field (if any)” so it will always update when we get a new value. When we select a new value from our color picker, we set the value to our field in FileMaker via the script passing a parameter from JavaScript. Once we set the field in FileMaker to store the value, our auto-enter fields update to the color we selected. Cool!
To check this and the other demos mentioned above, download the sample file below. I have attempted to keep it simple and easy to understand. I know I could do more to clean up the interface in these examples but have left it very bare-bones for you to examine.
Get the Demo File
Some other things to keep in mind when using web viewers:
- Your context is the current record. A web viewer loads for each record that you are on.
- In FM Go, you are limited to the number of web viewers on a layout.
- You should only have one copy/version of FileMaker (mostly for developers).
Of course, we are just scratching the surface of what you can do. Javascript is a great resource to add to your FileMaker tool belt, and now is a great time to add it!
Learn More About FileMaker Web Viewer and Javascript
- Best Practices for FileMaker Web Viewer Integrations
- How to Build A Pivot Table in FileMaker
- JavaScript Changes in FileMaker 16
- Creating JSON in FileMaker 16
- Parsing JSON in FileMaker 16
- Display Complex Web Viewers in WebDirect
- FileMaker and Javascript: AJAX POST
Leveraging Javascript in Your FileMaker Solution
Have a specific question or need? Our team builds custom web integrations with FileMaker to help businesses make their solutions more powerful. Contact us to learn more about what we can do for you.
References
- W3 Schools Javascript Tutorial http://www.w3schools.com/js/default.asp
- jQuery http://jquery.com/
I am hoping you may have a solution to a problem I am having. Using your instructions above (and other resources) I have created a layout that is meant to run on filemakerGo. It consists of a small web viewer that is running an elapsed time timer built in javascript. There is a start/stop button and a reset button and when the timer is stopped it passes the elapsed time into a script. It all works beautifully….except….I also have several pop-overs on this layout that each contain a web viewer. Once I have opened and closed a few of these web viewers the timer stops and resets.
I assume it is when I have exceeded my 5 or 6 web-viewers on a layout. I have tried programming various script steps to ‘go back’ to the timer once a pop-over is closed to try to make it the active one again but to no avail.
Any ideas?
Chris Andersson
Hi Chris, you may be running into a limitation of having too many web viewers. According to the documentation, this is dependent on the memory available in your device, with a max of 4.
Thanks for this little demo! Checked it out and it works like a charm on a mac. On Windows it won’t work. Interesting though: Using the complete string and adding it in the address field of ie11 while fmp is running returns the fmp screen with the script doing what it is supposed to … meaning it calls the script within fmp
Any ideas?
Hi Ernst,
I tested this in windows 7, and it worked fine for me. You might want to check that there are no other instances of filemaker running, that can possibly cause a conflict. Also that you are running the latest version of FMP.
I tried your first example in Filemaker Pro 13 Advanced using Windows 7, but what I get back is a pop-up window saying:
The file “Test1” could not be opened. Either the host is not availble, or the file is not available on that host.
Any suggestions?
Thanks for your article.
I have one instance each of Filemaker 12 Pro Advanced and 13 Pro Advanced.
I discovered that when I open the v12 instances before opening the v13, the javascript callback from the web viewer to fIlemaker doesn’t work on v13. It appears to be “talking” to the v12 instance because it comes in front of the window stack.
However, when I close the v12 or open the v12 after v13, it works.
Is there a way to make sure it works all the time?
Michael,
Having two versions of filemaker is likely to be a issue only if you are a developer, especially with Pro Advanced versions. The simplest answer is to be aware and launch FileMaker Pro 13 (any version) first.
URL Schemes are handled by the OS, which will use the application registered to handle the URL… http opens in web browsers, mailto opens in email clients, etc..
Hi Mike,
Wonderful article, thanks for writing it.
One question – what type of information can be passed to filemaker as a parameter? For example, I would like to pass an image from my Javascript code to a filemaker container field.
I am trying to set a variable in my code = to the toImage() function in javascript, and then using the fmp:// url, adding on my variable as a parameter as you laid out in your example – but when it comes accross it just shows up in my container as “undefined”.
I thought maybe I could pass it as encoded text maybe.
What are your thoughts?
Thanks again!
Great file to get me started…. You don’t by chance have an example of using Javascript to open a filemaker image in a JS enable image editor which then returns edited image back to FM ??
Hello Mr. Duncan, do you know if is it possible to use this technique in a Runtime? Because I tried with no success… Thank you
Giuseppe,
This won't work with runtimes, since the application registers itself with the OS as the handler for url schemes. The fmp protocol is specific to FileMaker Pro, either regular or advanced.
Thank you for delivering a valuable information.
Can I translate this article into Japanese and put it on my blog?
I see a possible issue with filemaker web view using IE on windows platforms. Under most normal security settings Internet Explorer restricts scripts (over a certain size it seems) from running. I think in most cases it is not possible to change the IE security settings for all users running the DB. This very much restricts filemaker from taking advantage of fun javascripting! Perhaps someone has come up with a workaround for this?
Question on this technique. I am new to filemaker, but I have been a web programmer for years, so this all makes pretty good sense to me. But one question I have. If I run a callback/ajax request to a filemaker script, how do I retrieve data from the script. Your examples show how to sent a parameter to the script. I am wondering how to get the script to pass data back to the web viewer in the same call.
Josh,
From FileMaker, you can set the html used in the webviewer to whatever you like, so you can reset with new content after getting a response back from the callback in javascript. It wouldn't necessarilly be in the same call, but could be part of the same script routine in FM. Would that work for you?
Hi There,
I am using the Google Maps JavaScript API v3 to bring up a custom map for routing between jobs.
On the map I have an info window that is populated via variables with client report and site information. What I would like to do is have a button in the infoWindow section of the map that takes me to the record relating to the data displayed in the infoWindow
Is this able to be achieved?
Hi Lloyd – did you resolve this? I’ve been researching the same as whilst it’s definitely possible (see examples below). I’m having issues getting this to work on my own Google Cluster Map. See examples below though.
– http://timdietrich.me/fmeasymaps/
-http://hbase.net/2012/02/17/creating-google-cluster-maps-from-filemaker/
Hi! Maybe I am missing something … but I am not able to see where you have reference the external jqeury colorpicker in the demo that you have provided. Could u please tell me where the reference is placed inside the demo … I want to use other jquery tools and not sure where to reference them in the filemaker file ….
Thanks
In this case, the HTML used is stored in a field. If you look at the field named “html1” in the Resources table/layout, you will see the references there to both Jquery and the picker. Thanks
Pingback: Click, Display, Capture – Google Map Longitude & Latitude into FileMaker Pro | HomeBase Software
Hi Mike, I have a piece of code works well with IE 9, but stopped working with IE 11. Could you tell why? Seems the saving function is not working:
“data:text/html,” &
”
#div2 {
width: 100%;
height: 100%;
}
Edit Target
Edit Target ” & Targets::Target Number & ” below
Save Changes
var currentFileName = ‘” &Get ( FileName) & Case ( fnGetFMMajorVersionNumber <= 13 ;".fmp12") & "';
$(function () {
jsdraw = createEmptyJSDraw(‘div2’);
var v = [];
$(‘#search-button’).on(‘click’, function () {
v.push(“& Targets::Target Number &”);
v.push(jsdraw.getSmiles());
v.push(jsdraw.getMolfile().replace(/[\n\r]/g, ‘|’));
hashcodeGetter(jsdraw.getSmiles(), function (d) {
v.push(d.hashcode);
var status = currentFileName+’:EditTarget:’+v.join(‘\r’);
window.status = status;
alert(window.status);
v = [];
});
});
});
“
Hi Hua,
The code you show here seems like it may be incomplete, if it came through ok. Some may have gotten lost when posting it.
It looks like it references some other javascript library that I do not see reference to. I any case, I often get the necessary code working in a standalone html file and debug in a browser, then get the working code back into FM.
Also, I think the web viewer may be more strict than general web browsers with well formed HTML with doctype definitions, so you might look at that as well.
Mike
Thank you for sharing! Keep up the great work!
Mike,
Based on the clever ideas and guidance you provided above, I have implemented a web viewer-based calendar solution for my personal use.
The solution feeds a calculated field full of CSS and HTML to a web viewer to see a monthly view for a selected month. For the monthly grid, the calculated code uses absolutely placed html div’s, and the events inside the div for a given day use FMP protocol based links to do things like bring the days events up for edit in a pop-over on the same layout as the calendar web viewer. This all works nicely in FileMaker – in fact its pretty awesome.
I am wondering about a way to make the calendar work in Web Direct (without a complete re-architecture not based on web viewers). As implemented, the solution doesn’t work in Web Direct since the FMP protocol links clicked inside the web viewer don’t have the desired affect inside the web direct session (in fact, they pull up FileMaker on my client computer and perform the linked script therein). I can see how I might use a logical switch to alternatively calculate FMP vs. HTML links on events – based on which platform the solution is being viewed in (FM vs. WD). I can’t figure out how to code the alternate link to trigger a series of events that would for instance, open a pop-over in the web direct session (as happens in FileMaker when using FMP protocol links). Does this make any sense? Any ideas?
To restate in less words: A data-statement link inside a web viewer inside a web direct session that can open a popover on the same layout that the web viewer is on (or similar).
Regards,
Ed
Hi Ed,
You are right that the FMP protocol only works for FM Pro or Go apps. There is a workaround for WebDirect, but involves several more moving pieces, but it is possible.
For WebDirect, instead of calling the FMP protocol, you can invoke some CWP that will get the information to FM Server. Getting the script to fire on the WebDirect users session is possible if you use the onRecordLoad script trigger to fire in another window by deleting a session record out from under the users session. I do this with a “session” record for each logged in user, and hiding that window so they do not see it. As long as the virtual window is open, the script trigger will still fire, and then you can perform a script that activates a popup, if that is what you want.
Mike
Is there a way to put a browser in full screen mode? New webdirect users often do not click the correct buttons in a filemaker solutions and do not log out properly. They often close the browser or use the back button of the browser. Putting the browser in full screen mode and disabling the F11 key may be a solution for this. The key is thus to put the browser in full screen mode using some script. (filemaker script, or javascript or a combination of the two.)
I do not think there is a reliable way of doing that, especially across all platforms. I would not recommend it anyway, as it could cause more confusion that it solves and disrupt other tabs they may have open. You could always show a tip screen for new users with additional instructions, and allow them to not show it again if they choose.
It is possible to open the chrome browser in full screen mode and go to a specific URL. But for this to work you need to have a shortcut file on the desktop that contains the instructions. The F11 key is disabled and it is only possible to close the chrome broser with alt F4. This is not an ideal solution, but the best one I found up to this moment. A script that can put the chrome browser in full screen mode would be a better solution. Webdirect should somehow put the chrome browser into full screen mode. The desktop shortcut does not work well if chrome is already open. Could it be possible to run some javascript code in a filemaker webviewer that puts chrome into full screen mode?
Not that I know of, javascript doesn’t target a specific application that way.
I know this thread is over a year old, but perhaps someone here can help me.
I have a similar situation in that:
I am bundling a FileMaker app in FIAS (iOS-SDK).
I use the web viewer to drag and drop images (records in FileMaker).
On FileMaker desktop (FM16) and in FileMaker Go on the iPad and iPhone, this works perfectly.
You can drag an image and it triggers a script in FileMaker to resort the records. But when I bundle the app in Xcode and attempt to drag an image in the web viewer — as an iOS bundled app — although the drag moves, it does not WRITE the change (sort number) back to FileMaker.┬áΓÇ¿
ΓÇ¿
The error in Xcode is: 
Could not signal service com.apple.WebKit.WebContent: 113: Could not find specified service
The Web Viewer content is:ΓÇ¿
“file:/” & If(Get(SystemPlatform)=1;”volumes”;””) ┬á& Get(TemporaryPath) & “sortByDrag.html”ΓÇ¿
ΓÇ¿
I know this is stretch, using FIAS to do this, but it works just fine in FM Go.
Perhaps something to do with the URL Scheme definition in Xcode??
ΓÇ¿
Any help would be appreciated,
Aaton
Unfortunately this will not work when using the iOS SDK to bundle an app. The fmp url will try to launch FileMaker, not your bundled app. And there is no way to register your app with the OS with a url prefix like “fmp:/” or anything else, so it is just not supported.
I’ve been taking a few baby steps experimenting with javascript and filemaker and I’ve come across a minor issue. Using the Get Form Input example I find that if the password field contains an equals sign the script failed. Is there a workaround?
Hi Chris, this was a simple example, but if you change the html code in the web viewer it can work. Where you see the fmp URL called, change “theParam” to “encodeURIComponent(theParam)” so it URL encodes the characters, then it can work.
Perfect thanks.
Pingback: JavascriptとFileMaker入門 | Not Only FileMaker
Pingback: FileMakerとJavascript:AJAX POST | Not Only FileMaker
Your color picker no longer seems to work. It just shows a small text box now instead of the color picker.. Not sure why this file no longer works? I’ve tried opening it in FM 16, 17 & 18 and cannot get it to show me a color picker…
The linked javascript from their github repo needed to use a different version of jquery. If you find that line and update it to “http://code.jquery.com/jquery-1.11.0.js” then it should work again. I have also updated the attached sample file if you want to download a new one. I also updated the security settings in the file to allow fmpurls in the privileges.
Dear Mike,
I want to pass on the x and y of scrolling to an apple script. I wrote a littel script in java which partially works. It passes on the string p but for x and y I only get a 0. Can you please help me to find out what I am doing wrong. I enjoyed reading your article. With your descriptions I am almost there, but still the 0 drives me crazy. Have a great day. Here is my script.
Thanks Peter
window.onscroll = function() {koordinate()};
function koordinate() {
x = document.body.scrollLeft;
y = document.body.scrollTop;
p = “x” + x + ” ” + “y” + y
theURL = “fmp://$/tablet\?script=sos¶m=” + p;
window.location = theURL ;
return false;
}
My guess would be the space in the script parameter. Can you test in your script to see what the entire script parameter is and maybe use a different delimiter in your param to get both values, or specify each as a separate variable.
Thanks. I did try it already. The transfer of the variable p is not the problem. I tested to sign a value to x or y and it was transfered perfectly. I found out that document.body.scrollLeft does not read the value. Can this be?
I am not familiar with that in particular, but it may need to be tested in safari (mac) and ie (win) to see if it supported.
Dear Mike,
I am dipping my toe into the javascript kiddie pool and I happened across this blog-post. I can’t get the Hello World example to work.
I’m running FMPA18 on a Mac (Catalina), and when I try to save the web-viewer code that you provided, I get
“The Specified Table cannot be found” error. When I click OK, FM highlights the code “fmp” in the tag.
What’s wrong. I feel like a goob unable to execute a Hello World example.
If you were copying text out of the blog post, I think wordpress may have taken out a couple backslashes that should have been in as escape characters. I tried to fix in the blog post, but you can also see it in the sample file. Hope that helps.